Kotlin is the latest JVM programming language from the JetBrains. Google has made it the official language for Android Development along with Java. Developers say it addresses the issues faced in Java programming. I have written a lot of Kotlin tutorials and here I am providing important kotlin interview questions.
Here I am providing Kotlin Interview Questions and Answers that will help you in your Kotlin Interviews. These Kotlin interview questions are good for beginners as well as experienced programmers. There are coding questions too to brush up your coding skills.
Java Virtual Machine(JVM) is the Target Platform of Kotlin. Kotlin is 100% interoperable with Java since both, on compilation produce bytecode. Hence Kotlin code can be called from Java and vice-versa.
There are two major differences between Java and Kotlin variable declaration:
The type of declaration In Java the declaration look like this:
String s = "Java String";
int x = 10;
In Kotlin the declaration looks like:
val s: String = "Hi"
var x = 5
In Kotlin, the declaration begins with a val
and a var
followed by the optional type. Kotlin can automatically detect the type using type inference.
Default value The following is possible in Java:
String s:
The following variable declaration in Kotlin is not valid.
val s: String
val
variables cannot be changed. They’re like final modifiers in Java. A var
can be reassigned. The reassigned value must be of the same data type.
fun main(args: Array<String>) {
val s: String = "Hi"
var x = 5
x = "6".toInt()
}
We use the toInt()
method to convert the String to an Int.
Kotlin puts a lot of weight behind null safety which is an approach to prevent the dreaded Null Pointer Exceptions by using nullable types which are like String?
, Int?
, Float?
etc. These act as a wrapper type and can hold null values. A nullable value cannot be added to another nullable or basic type of value. To retrieve the basic types we need to use safe calls that unwrap the Nullable Types. If on unwrapping, the value is null we can choose to ignore or use a default value instead. The Elvis Operator is used to safely unwrap the value from the Nullable. It’s represented as ?:
over the nullable type. The value on the right hand side would be used if the nullable type holds a null.
var str: String? = "JournalDev.com"
var newStr = str?: "Default Value"
str = null
newStr = str?: "Default Value"
const
? How does it differ from a val
?By default val
properties are set at runtime. Adding a const modifier on a val
would make a compile-time constant. A const
cannot be used with a var
or on its own. A const
is not applicable on a local variable.
At the language level, we cannot use the above-mentioned types. But the JVM bytecode that’s compiled does certainly have them.
The main
function is the entry point of every Kotlin program. In Kotlin we can choose not to write the main function inside the class. On compiling the JVM implicitly encapsulates it in a class. The strings passed in the form of Array<String>
are used to retrieve the command line arguments.
!!
different from ?. in unwrapping the nullable values? Is there any other way to unwrap nullable values safely?!!
is used to force unwrap the nullable type to get the value. If the value returned is a null, it would lead to a runtime crash. Hence a !!
operator should be only used when you’re absolutely sure that the value won’t be null at all. Otherwise, you’ll get the dreaded null pointer exception. On the other hand, a ?. is an Elvis Operator that does a safe call. We can use the lambda expression let
on the nullable value to unwrap safely as shown below. Here the let expression does a safe call to unwrap the nullable type.
fun sumOf(a: Int, b: Int): Int{
return a + b
}
A function’s return type is defined after the :
Functions in Kotlin can be declared at the root of the Kotlin file.
\== is used to compare the values are equal or not. === is used to check if the references are equal or not.
- public
- internal
- protected
- private
`public` is the default visibility modifier.
```
class A{
}
class B : A(){
}
```
**NO**. By default classes are final in Kotlin. To make them non-final, you need to add the `open` modifier.
```
open class A{
}
class B : A(){
}
```
Constructors in Kotlin are of two types: **Primary** - These are defined in the class headers. They cannot hold any logic. There's only one primary constructor per class. **Secondary** - They're defined in the class body. They must delegate to the primary constructor if it exists. They can hold logic. There can be more than one secondary constructors.
```
class User(name: String, isAdmin: Boolean){
constructor(name: String, isAdmin: Boolean, age: Int) :this(name, isAdmin)
{
this.age = age
}
}
```
`init` is the initialiser block in Kotlin. It's executed once the primary constructor is instantiated. If you invoke a secondary constructor, then it works after the primary one as it is composed in the chain.
String interpolation is used to evaluate string templates. We use the symbol $ to add variables inside a string.
```
val name = "Journaldev.com"
val desc = "$name now has Kotlin Interview Questions too. ${name.length}"
```
Using `{}` we can compute an expression too.
By default, the constructor arguments are `val` unless explicitly set to `var`.
**NO**. Unlike Java, in Kotlin, new isn't a keyword. We can instantiate a class in the following way:
```
class A
var a = A()
val new = A()
```
when is the equivalent of `switch` in `Kotlin`. The default statement in a when is represented using the else statement.
```
var num = 10
when (num) {
0..4 -> print("value is 0")
5 -> print("value is 5")
else -> {
print("value is in neither of the above.")
}
}
```
`when` statments have a default break statement in them.
In Java, to create a class that stores data, you need to set the variables, the getters and the setters, override the `toString()`, `hash()` and `copy()` functions. In Kotlin you just need to add the `data` keyword on the class and all of the above would automatically be created under the hood.
```
data class Book(var name: String, var authorName: String)
fun main(args: Array<String>) {
val book = Book("Kotlin Tutorials","Anupam")
}
```
Thus, data classes saves us with lot of code. It creates component functions such as `component1()`.. `componentN()` for each of the variables. [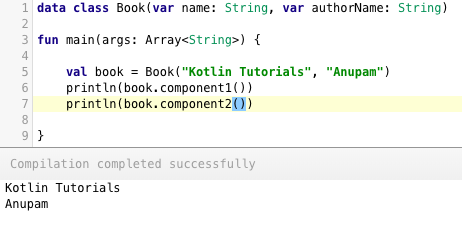](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-data-classes.png)
Destructuring Declarations is a smart way to assign multiple values to variables from data stored in objects/arrays. [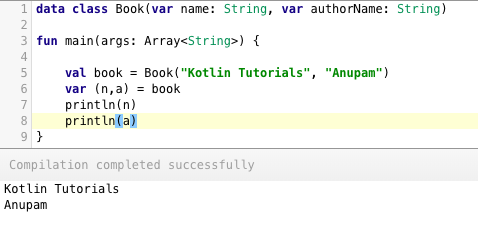](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-destructuring-declarations.png) Within paratheses, we've set the variable declarations. Under the hood, destructuring declarations create component functions for each of the class variables.
[Inline functions](/community/tutorials/kotlin-inline-function-reified) are used to save us memory overhead by preventing object allocations for the anonymous functions/lambda expressions called. Instead, it provides that functions body to the function that calls it at runtime. This increases the bytecode size slightly but saves us a lot of memory. [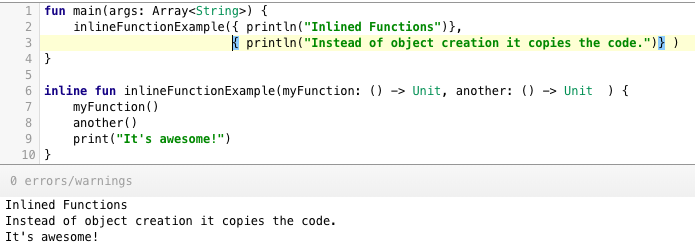](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-inline-functions.png) [infix functions](/community/tutorials/kotlin-functions) on the other are used to call functions without parentheses or brackets. Doing so, the code looks much more like a natural language. [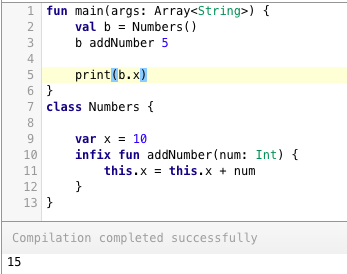](https://journaldev.nyc3.cdn.digitaloceanspaces.com/2018/04/kotlin-interview-questions-infix-notations.png)
Both are used to delay the property initializations in Kotlin `lateinit` is a modifier used with var and is used to set the value to the var at a later point. `lazy` is a method or rather say lambda expression. It's set on a val only. The val would be created at runtime when it's required.
```
val x: Int by lazy { 10 }
lateinit var y: String
```
To use the singleton pattern for our class we must use the keyword `object`
```
object MySingletonClass
```
An `object` cannot have a constructor set. We can use the init block inside it though.
**NO**. Kotlin doesn't have the static keyword. To create static method in our class we use the `companion object`. Following is the Java code:
```
class A {
public static int returnMe() { return 5; }
}
```
The equivalent Kotlin code would look like this:
```
class A {
companion object {
fun a() : Int = 5
}
}
```
To invoke this we simply do: `A.a()`.
```
val arr = arrayOf(1, 2, 3);
```
The type is Array<Int>.
That’s all for Kotlin interview questions and answers.
Thanks for learning with the DigitalOcean Community. Check out our offerings for compute, storage, networking, and managed databases.
I think there is an error on number 18: var num = 10 when (num) { 0…4 -> print(“value is 0”) 5 -> print(“value is 5”) else -> { print(“value is in neither of the above.”) } } Surely for the line 0…4 the value would be from 1-4? But the print says the value is 0, which also makes the else print statement below that incorrect
- Aaron Finn
Check question 14. -init is the intialiser block in Kotlin. It’s executed once the constructor is instantiated. Statement from Kotlin docs -Delegation to the primary constructor happens as the Drst statement of a secondary constructor, so the code in all initializer blocks is executed before the secondary constructor body. Even if the class has no primary constructor, the delegation still happens implicitly, and the initializer blocks are still executed: Please update the answer, Thanks,
- Shashikant Sule
The answer to question 8 is not right about let: 1 for null safety use not str.let{…} but str?.let{…} 2 inside the body of let’s parameter we use `it` not `str` See https://www.journaldev.com/19467/kotlin-let-run-also-apply-with#let-for-null-checks
- Mihai Manole
hi i will have interview. would u mind giving me more reference? its impossible they will ask basic question thanks.
- lemert gie
Get paid to write technical tutorials and select a tech-focused charity to receive a matching donation.
Full documentation for every DigitalOcean product.
The Wave has everything you need to know about building a business, from raising funding to marketing your product.
Stay up to date by signing up for DigitalOcean’s Infrastructure as a Newsletter.
New accounts only. By submitting your email you agree to our Privacy Policy
Scale up as you grow — whether you're running one virtual machine or ten thousand.
Sign up and get $200 in credit for your first 60 days with DigitalOcean.*
*This promotional offer applies to new accounts only.